Last weekend I had my first major in-person conference since the SARS-2 pandemic began: about 150 people congregated from all over Germany to quarrel and, more importantly, to settle quarrels. But it's still Corona, and thus the organisers put in place a whole bunch of disease control measures. A relatively minor of these was monitoring the CO2 levels in the conference hall as a proxy for how much aerosol may have accumulated. The monitor devices they got were powered by USB, and since I was sitting on the stage with a computer having USB ports anyway, I was asked to run (and keep an eye on) the CO2 monitor for that area.
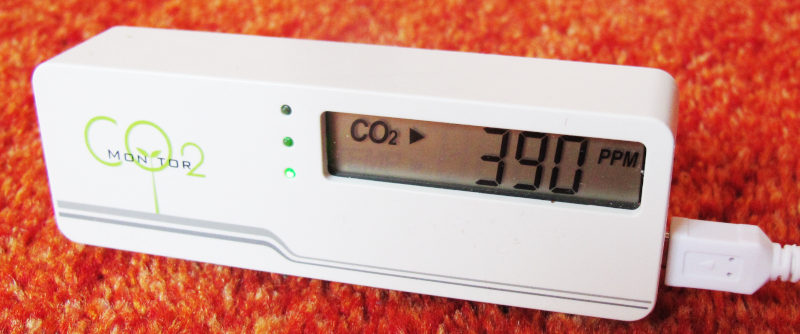
The CO2 sensor I got my hands on. While it registers as a Holtek USB-zyTemp, on the back it says “TFA Dostmann Kat.Nr. 31.5006.02“. I suppose the German word for what's going on here is “Wertschöpfungskette“ (I'm not making this up. The word, I mean. Why there are so many companies involved I really can only guess).
When plugging in the thing, my syslog[1] intriguingly said:
usb 1-1: new low-speed USB device number 64 using xhci_hcd usb 1-1: New USB device found, idVendor=04d9, idProduct=a052, bcdDevice= 2.00 usb 1-1: New USB device strings: Mfr=1, Product=2, SerialNumber=3 usb 1-1: Product: USB-zyTemp usb 1-1: Manufacturer: Holtek usb 1-1: SerialNumber: 2.00 hid-generic 0003:04D9:A052.006B: hiddev96: USB HID v1.10 Device [Holtek USB-zyTemp] on usb-0000:00:14.0-1/input0 hid-generic 0003:04D9:A052.006C: hiddev96: USB HID v1.10 Device [Holtek USB-zyTemp] on usb-0000:00:14.0-1/input0
So: The USB is not only there for power. The thing can actually talk to the computer. Using the protocol for human interface devices (HID, i.e., keyboards, mice, remote controls and such) perhaps is a bit funky for a measurement device, but, on closer reflection, fairly reasonable: just as the mouse reports changes in its position, the monitor reports changes in CO2 levels and temperatures of the air inside of it.
Asking Duckduckgo for the USB id "04d9:a052" (be sure to make it a phrase search with the quotes our you'll be bombarded by pages on bitcoin scams) yields a blog post on decrypting the wire protocol and, even better, a github repo with a few modules of Python to read out values and do all kinds of things with them.
However, I felt like the amount of code in that repo was a bit excessive for something that's in the league of what I call a classical 200 lines problem – meaning: a single Python script that works without any sort of installation should really do –, since all I wanted (for now) was a gadget that shows the current values plus a bit of history.
Hence, I explanted and streamlined the core readout code and added some 100 lines of Tkinter to produce co2display.py3, showing an interface like this:
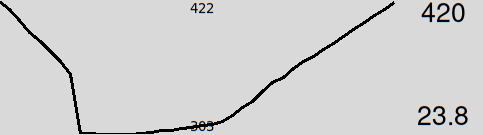
This is how opening a window (the sharp drop of the curve on the left), then opening a second one (the even sharper drop following) and closing it again while staying in the room (the gentle slope on the right) looks like in co2display.py. In case it's not obvious: The current CO2 concentration was 420 ppm, and the temperature 23.8 degrees Centigrade (where I'm sure the thing doesn't measure to tenths of Kelvins; but then who cares about thenths of Kelvins?) when I took that screenshot.
If you have devices like the zyTemp yourself, you can just download the program, install the python3-hid package (or its equivalent on non-Debian boxes) and run it; well, except that you need to make sure you can read the HID device nodes as non-root. The easiest way to do that is to (as root) create a file /etc/udev/rules.d/80-co2meter.rules containing:
ATTR{idVendor}=="04d9", ATTR{idProduct}=="a052", SUBSYSTEM=="usb", MODE:="0666"
This udev rule simply says that whenever a device with the respective ids is plugged in, any device node created will be world-readable and world-writable (and yeah, it does over-produce a bit[2]).
After adding the rule, unplug and replug the device and then type python3 co2display.py3. Ah, yes, the startup (i.e., the display until actual data is available) probably could do with a bit of extra polish.
First Observations
I'm rather intrigued by the dynamics of CO2 levels measured in that way (where I've not attempted to estimates errors yet). In reasonably undisturbed nature at the end of the summer and during the day, I've seen 250 to 280 ppm, which would be consistent with mean global pre-industrial levels (which Wikipedia claims is about 280 ppm). I'm curious how this will evolve towards winter and next spring, as I'd guess Germany's temporal mean will hardly be below the global one of a bit more than 400 (again according to Wikipedia).
In a basically empty train I've seen 350 ppm yesterday, a slightly stuffy train about 30% full was at 1015 ppm, about as much as I have in my office after something like an hour of work (anecdotically, I think half an hour of telecon makes for a comparable increase, but I can hardly believe that idle chat causes more CO2 production than heavy-duty thinking. Hm).
On a balcony 10 m above a reasonably busy road (of order one car every 10 seconds) in a lightly built-up area I saw 330 ppm under mildly breezy conditions, dropping further to 300 as the wind picked up. Surprisingly, this didn't change as I went down to the street level. I can hardly wait for those winter days when the exhaust gases are strong in one's nose: I cannot imagine that won't be reflected in the CO2.
The funkiest measurements I made on the way home from the meeting that got the device into my hands in the first place, where I bit the bullet and joined friends who had travelled their in a car (yikes!). While speeding down the Autobahn, depending on where I measured in the small car (a Mazda if I remember correctly) carrying four people, I found anything from 250 ppm near the ventilation flaps to 700 ppm around my head to 1000 ppm between the two rear passengers. And these values were rather stable as long as the windows were closed. Wow. Air flows in cars must be pretty tightly engineered.
Technics
If you look at the program code, you'll see that I'm basically polling the device:
def _update(self): try: self._take_sample() ... finally: self.after(self.sample_interval, self._update)
– that's how I usually do timed things in tkinter programs, where, as normal in GUI programming, there's an event loop external to your code and you cannot just say something like time.wait() or so.
Polling is rarely pretty, but it's particularly inappropriate in this case, as the device (or so I think at this point) really sends data as it sees fit, and it clearly would be a lot better to just sit there and wait for its input. Additionally, _take_sample, written as it is, can take quite a bit of time, and during that time the UI is unresponsive, which in this case means that resizes and redraws don't take place.
That latter problem could easily be fixed by pushing the I/O into a thread. But then this kind of thing is what select was invented for, or, these days, wrappers for it (or rather its friends) usually subsumed under “async programming“.
However, marrying async and the Tkinter event loop is still painful, as evinced by this 2016 bug against tkinter. It's still open. Going properly async on the CO2monitor class in the program will still be the next thing to do, presumably using threads.
Ah, that, and recovering from plugging the device out and in again, which would also improve behaviour as people suspend the machine.
Apart from that, there's just one detail I should perhaps highlight in the code: The
self.bind("<Configure>", lambda ev: self._update_plot())
in the constructor. That makes the history plot re-scale if the UI is re-sized, and I've always found it a bit under-documented that <Configure> is the event to listen for in this situation. But perhaps that's just me.
Nachtrag (2021-10-19)
I've updated co2display.py3 as published here, since I've been hacking on it quite a bit in the meantime. In particular, if you rename the script co2log.py (or anything else with “log” in it), this will run as a plain logger (logging into /var/log/co2-levels by default), and there's a systemd unit at the end of the script that lets you run this automatically; send a HUP to the process to make it re-open its log; this may be useful together with logrotate if you let this run for weeks your months.
You can also enable logging while letting the Tk UI run by passing a -d option, and you can adjust the interval in which log values are written or plotted using -i.
The citing article(s) listed below discuss a few things I've found out with the device (albeit in German).
[1] | One of the more important parts of my desktop setup is starting: LC_ALL=de_DE root-tail --shade -f -g 752x350+614-0 \ -fn lucidasanstypewriter-bold-8 /var/log/syslog as the X server starts up. With this (at least as long as systemd's journald doesn't take over entirely), the syslog is in a corner of the desktop background that I tend to keep uncovered unless I'm really starved for screen space, and I have a good chance of noticing distress signals the machine might send. It's also easy to view its chit-chat when I want to, as I usually do when I'm plugging anything in, ranging from ethernet cables (is there a carrier on it?) to power supplies (does it carry power?) to memory sticks (what partitions are on it?). |
[2] | For instance, ACTION=="add" would probably prevent the rule from firing when it's not needed, but my advice with udev rules is to not try to be smart; my attempts in that direction have rather consistentyl tended to lead to a lot of puzzling about why a rule either doesn't fire or fires too often, and whenever I had a clever scheme it broke with a kernel upgrade one to five years later. Little wonder: Udev constraints in the end are expressed in terms of traversals of the /sys directory tree, and tree traversal – things executed by pushdown automata, if you will – tend to be a challenge to the human mind. As opposed to, by the way, Turing machines on the one side, for which we're hopeless unless aided by large amounts of heavy-duty math, and finite state machines – a.k.a. regular expressions – on the other side, which most people can usually figure out easily (until they outgrow our memories). Anyway: I've had rules with this pattern on my box since, what, 15 years, and I've never had to maintain them. To me, that's more than enough to justify the waste of a few CPU cycles. |
Zitiert in: 'Failed to reset ACL' with elogind: Why? Neun Monate Umwelt-CO₂, Teil II: Hochpass, Tiefpass, Spektrum Neun Monate Umwelt-CO2, Teil I: Taugen die Daten? Schrödingers Pandemie 2: Unsere Kraft ist die Gewerkschaft Schrödingers Pandemie 'Failed to reset ACL' with elogind: Why? Eine Sturmfront zieht durch Kohlendioxid auf dem Balkon Kohlendioxid und die thermische Leistung von Menschen